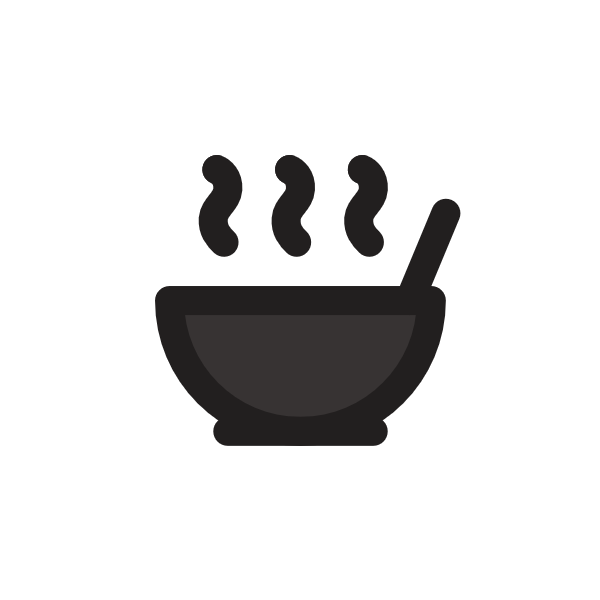
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Return-oriented programming
Read the original article here.
The Forbidden Code: Return-Oriented Programming
Welcome to "The Forbidden Code," where we delve into the techniques that push the boundaries of software and challenge conventional security measures. In this chapter, we explore Return-Oriented Programming (ROP), a powerful and sophisticated exploit technique that allows attackers to execute arbitrary code even on systems protected by advanced security defenses like executable-space protection. While often taught in advanced security courses, mastering ROP involves understanding low-level system mechanics in ways most mainstream programming education avoids. It's a true underground technique for the discerning digital architect.
Understanding the Landscape: From Simple Bugs to Sophisticated Exploits
Before diving into ROP, it's essential to understand the evolution of code execution attacks and the defenses developed to counter them. ROP didn't appear in a vacuum; it arose as a direct response to security measures designed to stop simpler attacks.
The Foundation: Buffer Overflows
Many low-level code execution techniques, including ROP, begin with a common vulnerability: the buffer overflow.
Definition: Buffer Overflow A buffer overflow occurs when a program attempts to write more data to a fixed-size memory buffer than it can hold. If this buffer is located on the call stack, the excess data can overwrite adjacent data, including critical control information like the return address.
Think of a buffer as a small box intended to hold a specific number of items. If you try to cram more items into the box than it can fit, they spill out. In memory, this "spill" can overwrite data immediately following the buffer. If that data is the return address – the pointer telling the function where to go after it finishes – an attacker can redirect the program's execution flow.
Early Exploitation: Injecting Code on the Stack
In the early days, exploiting a buffer overflow was relatively straightforward. An attacker would:
- Find a buffer overflow vulnerability, typically in a function handling user input.
- Craft input data that first overflowed the buffer and then wrote malicious code (the "payload") onto the stack, usually just after the buffer.
- Overwrite the function's return address with the memory address where the injected payload code was placed on the stack.
- When the vulnerable function finished, instead of returning to its legitimate caller, it would jump to and execute the attacker's payload code.
This was the classic "stack smashing" attack. It was simple, effective, and rampant before significant operating system defenses were implemented.
Example Scenario:
Imagine a C function char buffer[64]; gets(buffer);
. The gets()
function reads input until a newline or EOF, with no size limit check. If a user inputs more than 63 characters, the excess bytes overflow buffer
on the stack, potentially overwriting local variables, saved registers, and crucially, the return address. An attacker could supply 64 bytes of garbage to fill the buffer, followed by their malicious shellcode, and then the address of their shellcode.
The Defense Rises: Executable-Space Protection (DEP)
As stack smashing became common, operating systems introduced a crucial defense: Executable-Space Protection (ESP), also widely known as Data Execution Prevention (DEP).
Definition: Data Execution Prevention (DEP) A security feature that marks certain areas of memory as non-executable. Memory regions typically used for data storage (like the stack and heap) are marked non-executable. This prevents attackers from placing malicious code in these areas and then jumping to execute it.
With DEP enabled, the previous attack fails. The attacker can still overwrite the return address via a buffer overflow, but if they point it to their injected code on the stack, the processor will refuse to execute instructions from that memory region, typically resulting in a crash. This defense significantly hampered traditional stack smashing.
The Evolution to ROP: Working With What You Have
DEP forced attackers to get creative. Since they couldn't execute their own code injected into data segments, they had to find a way to execute code that already existed in memory marked as executable – which is typically the program's own code and the code of linked libraries (like the C standard library, libc
).
Step 1: Return-into-Library (Return-to-libc)
The first major evolutionary step was Return-into-Library, often specifically called Return-to-libc.
Definition: Return-into-Library (Return-to-libc) An exploit technique where an attacker uses a buffer overflow to overwrite a function's return address with the entry point of an existing library function (like
system()
fromlibc
). The attacker also manipulates the stack to place the arguments required by that library function immediately after the overwritten return address, according to the function's calling convention.
Instead of pointing the return address to injected shellcode, the attacker pointed it to a useful function already present in the program's address space (e.g., system("sh")
on Linux or ExitProcess(0)
on Windows). The attacker would then carefully craft the bytes immediately following the overwritten return address on the stack to mimic the arguments the chosen library function expected, followed by a fake return address (or the address of another function to call next).
Example Use Case: Using system("/bin/sh")
to spawn a shell on a Linux system. The attacker overflows a buffer, overwrites the return address with the address of the system()
function in libc
, places the string "/bin/sh" somewhere in memory (perhaps within the overflowed buffer itself or another writable location), and then places the address of "/bin/sh" on the stack where the first argument to system()
is expected according to the calling convention.
This technique successfully bypassed DEP because it only executed code already marked as executable (the library function). However, it had limitations:
- It relied on finding suitable entire library functions.
- It was highly dependent on specific function signatures and calling conventions, which changed (especially with the move to 64-bit architectures).
- Library developers started removing or restricting "dangerous" functions.
Step 2: Borrowed Code Chunks
The move to 64-bit x86 architectures introduced new calling conventions (like System V AMD64 ABI, common on Linux), where the first few function arguments are passed in registers instead of solely on the stack. This made simple Return-to-libc harder, as you couldn't just place arguments directly on the stack below the return address.
Attackers needed a way to get values into registers. This led to techniques that used small sequences of existing instructions ending in a return, primarily functions that would pop
values off the stack into registers. By chaining these "chunks," an attacker could load desired values into registers and then call a function using the correct calling convention.
This was a significant step, using parts of functions rather than just their entry points. It paved the way for full ROP.
The Dawn of ROP: Turing Completeness with Found Code
Return-oriented programming takes the concept of "borrowed code chunks" to its logical conclusion. Instead of just using sequences to set up a single function call, ROP chains many small instruction sequences, each ending in a return instruction, to perform arbitrary computations.
Definition: Return-Oriented Programming (ROP) A computer security exploit technique where an attacker gains control of the call stack to hijack program control flow and execute carefully chosen machine instruction sequences, called "gadgets," that are already present in the machine's memory. Each gadget typically ends in a return instruction. By chaining these gadgets together using a crafted stack, an attacker can make the program perform operations far beyond a single function call, potentially achieving Turing-complete functionality.
The core idea is to find existing, legitimate instruction sequences within the executable memory (the program itself, shared libraries, etc.) that perform small, useful operations (like moving data between registers, performing arithmetic, loading values from memory, performing system calls, etc.). These sequences are called gadgets.
Definition: Gadget (in ROP) A short sequence of machine instructions within an existing executable binary (program code or libraries) that performs a specific operation and ends with a control flow instruction, most commonly a
ret
(return) instruction. Gadgets are the building blocks of a ROP chain.
How ROP Works: The Stack as a Program
- Find the Vulnerability: The attack typically starts with a vulnerability that allows an attacker to control the content of the call stack, such as a buffer overflow.
- Locate Gadgets: The attacker analyzes the target binary (or its linked libraries) to find useful gadgets. These are sequences of instructions ending in
ret
. On architectures like x86, the variable instruction length and dense opcode space make it likely that even random byte sequences can be interpreted as valid instructions, increasing the pool of potential gadgets. Tools exist to automate this search. - Build the ROP Chain: The attacker crafts a sequence of memory addresses. This sequence is written onto the stack where the return address would normally be stored.
- The first address is the start of the first gadget.
- Subsequent addresses are placed on the stack to be popped off by the
ret
instructions of preceding gadgets.
- Trigger the Exploit: The vulnerable function returns. Instead of returning to its caller, it jumps to the address at the top of the manipulated stack – the first gadget's address.
- Chain Execution: The first gadget executes its instructions. When it hits its
ret
instruction, it pops the next address off the stack and jumps to that address, which is the start of the second gadget. This process repeats, executing the instructions of each gadget in the sequence.
Example Stack Layout (Conceptual):
[ ... function variables ... ]
[ Overwritten Return Address -> Address of Gadget 1 ]
[ Value to be used by Gadget 1 (popped into a register) ]
[ Address of Gadget 2 ]
[ Argument 1 for Gadget 2's operation ]
[ Argument 2 for Gadget 2's operation ]
[ Address of Gadget 3 ]
[ ... and so on ... ]
Each ret
instruction effectively acts as a "call" to the next address on the stack, while also handling stack cleanup (incrementing the stack pointer). By carefully selecting gadgets, the attacker can perform operations like:
- Loading arbitrary values into registers (
pop <reg>; ret
) - Moving data between registers (
mov <reg1>, <reg2>; ret
) - Performing arithmetic/logic operations (
add <reg1>, <reg2>; ret
) - Reading from or writing to memory (
mov <mem>, <reg>; ret
,mov <reg>, <mem>; ret
) - Performing system calls (
syscall; ret
or equivalent depending on architecture/OS).
By chaining these primitive operations, attackers can construct complex logic, effectively writing a new "program" using only pre-existing code snippets. Hovav Shacham demonstrated in 2007 that with a sufficiently large codebase (like the C standard library), enough gadgets exist to achieve Turing completeness – meaning, theoretically, any computation possible on a standard computer can be performed via ROP.
Example Gadget Sequence (Conceptual x86-64):
Suppose an attacker wants to call execve("/bin/sh", ["/bin/sh", NULL], NULL)
to get a shell. On Linux x86-64, this system call requires specific values in registers:
rax
= syscall number forexecve
(e.g., 59)rdi
= pointer to the string "/bin/sh"rsi
= pointer to the array["/bin/sh", NULL]
rdx
= pointer to the environment array (usually NULL)
The attacker would build a ROP chain on the stack like this:
- Address of
pop rax; ret
gadget - Value 59 (for
rax
) - Address of
pop rdi; ret
gadget - Address of "/bin/sh" string (attacker must ensure this string exists in memory, perhaps written via the overflow itself)
- Address of
pop rsi; ret
gadget - Address of the array
["/bin/sh", NULL]
- Address of
pop rdx; ret
gadget - Value 0 (for
rdx
) - Address of
syscall; ret
gadget
When the vulnerable function returns, it jumps to pop rax; ret
. This gadget pops 59 into rax
and returns, jumping to the next address on the stack (pop rdi; ret
). This continues until all registers are set. Finally, the syscall; ret
gadget is reached, executing execve
with the controlled arguments.
Why ROP is Powerful (and "Forbidden")
ROP is powerful because:
- It bypasses DEP by using only executable memory.
- It can bypass ASLR (Address Space Layout Randomization) if even a single address in a library can be leaked or guessed (since relative offsets between gadgets in that library remain constant). Brute-forcing 32-bit ASLR is feasible; 64-bit is harder but still potentially vulnerable to info leaks.
- It's highly flexible due to Turing completeness; attackers aren't limited to calling a single pre-defined function.
- It's difficult to distinguish ROP execution from legitimate program flow using simple checks, as it's executing valid instructions.
It's "forbidden" in the context of mainstream programming education because it's a technique primarily associated with exploiting vulnerabilities and achieving unauthorized access. Understanding ROP requires a deep dive into assembly language, memory layouts, calling conventions, and operating system internals – knowledge crucial for both offensive and defensive security, but not typically covered in standard development curricula.
ROP Variations
Attackers continuously innovate. One notable variation is ROP that doesn't rely solely on the ret
instruction.
ROP Without ret
(Jump-Oriented Programming - JOP)
Definition: Jump-Oriented Programming (JOP) A technique similar to ROP, but instead of relying on
ret
instructions to chain gadgets via the stack, it uses other control-flow instructions likejmp
orcall
to chain gadgets. The target addresses for these jumps or calls are often loaded into registers or derived from controlled data.
This technique is sometimes called "returnless ROP" or specifically JOP. On architectures like x86, sequences like pop <reg>; jmp <reg>
can effectively behave like a return by popping the next address off the stack into a register and then jumping to it. On ARM, sequences involving ldr pc, [sp]
followed by add sp, sp, #4
could mimic a return.
This variation makes ROP harder to detect for defenses that specifically monitor ret
instructions. However, it requires finding suitable instruction sequences that end in jmp
or call
and correctly manipulate control flow.
The Security Arms Race: Defenses Against ROP
Just as attackers evolved from simple buffer overflows to ROP, defenders have developed increasingly sophisticated countermeasures.
Address Space Layout Randomization (ASLR) Revisited
While ASLR makes predicting the absolute addresses of code difficult, it's often not a complete defense against ROP, as discussed.
Definition: Address Space Layout Randomization (ASLR) A computer security technique that involves randomly arranging the positions of key data areas, typically including the base of the executable, libraries, stack, and heap, in a process's address space. This makes it harder for an attacker to predict target addresses for jumps or calls (like gadget locations in ROP).
Limitations of ASLR:
- 32-bit ASLR: Limited address space means fewer bits for randomization, making brute force attacks sometimes feasible within minutes.
- Information Leakage: Any vulnerability that allows an attacker to read any memory address within a randomized module (like a library) can reveal its base address, allowing the attacker to calculate the addresses of all other gadgets within that module.
- Partial Overwrites: Sometimes an overflow only allows overwriting part of an address, or the attacker can control other memory locations.
- No-Randomization Modules: Some legacy code or specific program configurations might disable ASLR for certain modules.
More Advanced Randomization Techniques
Beyond ASLR, more drastic randomization techniques exist:
- Fine-grained ASLR: Randomizing not just library bases, but individual functions or even basic blocks (small sequences of instructions). This is harder to implement and can incur performance overhead.
- Binary Code Randomization / Shuffling: Techniques applied at build time or deployment time (e.g., in Cloud functions) that change the layout and even instruction sequences slightly for each instance of a program. This dramatically reduces the chance that an attacker's carefully crafted ROP chain for one instance will work on another, as gadget addresses change significantly. However, this makes testing difficult (you can't test every variation) and can impact performance.
Control Flow Integrity (CFI)
CFI is a broad category of defenses aiming to ensure that program execution follows a valid path determined at compile time. Specific CFI implementations target indirect branches (like returns, indirect jumps, and virtual calls) which are the entry points for ROP gadgets.
- kBouncer: This technique, implemented in the OS kernel, verifies that any return instruction (
ret
) transfers control flow back to a location immediately following acall
instruction. This explicitly breaks ROP chains, which jump from oneret
to an arbitrary gadget start, not necessarily after acall
. However, it has performance overhead and doesn't protect against JOP (which usesjmp
instead ofret
). - G-Free: A more comprehensive approach that aims to eliminate or protect all "free branch" instructions (like
ret
orcall
used indirectly) that attackers could hijack. It can involve compiler modifications to insert checks or obfuscate control flow targets. It may also protect return addresses using techniques similar to stack canaries.
Compiler-Based Defenses
Compilers can be modified to make ROP harder:
- Eliminating Gadgets: Techniques have been proposed to analyze code during compilation and modify instruction sequences to remove potential ROP gadgets. For example, replacing
ret
instructions with an indirect jump through a verified table of legitimate return addresses can break ROP chaining. - Instrumenting Code: Compilers can insert code that checks the validity of control flow targets at runtime.
Hardware-Assisted Defenses
Modern processor architectures are starting to include hardware features specifically designed to combat ROP and JOP:
- Pointer Authentication Codes (PAC): Introduced in ARMv8.3-A. This feature uses cryptography to "sign" pointers (like return addresses) before they are pushed onto the stack. When the pointer is read from the stack just before being used (e.g., by a
ret
instruction), the signature is verified using a secret key and context (like the current stack pointer). If the pointer or stack pointer has been tampered with, the verification fails, causing an exception. This makes overwriting return addresses without knowing the secret key extremely difficult. While strong, even PACs have been subject to side-channel attacks (like PACMAN). - Branch Target Identification (BTI): Introduced in ARMv8.5-A. This feature marks valid targets for indirect branches (
jmp
,call
,ret
used indirectly) with a special instruction (BTI
). Guarded memory pages (containing code intended to be protected) will fault if an indirect branch lands on an instruction that is not aBTI
instruction. Since ROP gadgets can start anywhere, the vast majority (often 99%+) will not start with aBTI
, causing the attack to fail on the first gadget jump. BTI and PAC are complementary: PAC protects the source pointer of the branch, BTI protects the destination.
These hardware defenses, while not yet ubiquitous, represent a significant step in raising the bar against ROP and related control-flow hijacking attacks.
Structured Exception Handler Overwrite Protection (SEHOP)
While primarily aimed at a specific type of stack overflow that targets the Structured Exception Handler chain on Windows, SEHOP is worth mentioning in this context. It helps protect against overwriting the pointers in the exception handler chain, which could otherwise be used in a way somewhat analogous to Return-to-libc to divert execution. However, SEHOP is not a direct defense against ROP chains built on function return addresses.
Conclusion: The Forbidden Code Revealed
Return-Oriented Programming is a prime example of how attackers adapt to defenses. Starting from simple buffer overflows, the cat-and-mouse game with security professionals led to increasingly sophisticated techniques. ROP bypasses fundamental defenses like DEP by treating existing code snippets as an instruction set, orchestrated via the stack. Its power lies in achieving arbitrary code execution (Turing completeness) through careful chaining of these "gadgets."
Understanding ROP is crucial for anyone serious about low-level security, whether for finding vulnerabilities or defending against them. It highlights the importance of robust vulnerability patching (especially buffer overflows), layered defenses (like ASLR and CFI), and keeping up with hardware-assisted security features. While ROP might seem like an "underground" programming technique, its principles are fundamental to understanding control flow hijacking and the ongoing battle for control of program execution in memory.
Related Articles
See Also
- "Amazon codewhisperer chat history missing"
- "Amazon codewhisperer keeps freezing mid-response"
- "Amazon codewhisperer keeps logging me out"
- "Amazon codewhisperer not generating code properly"
- "Amazon codewhisperer not loading past responses"
- "Amazon codewhisperer not responding"
- "Amazon codewhisperer not writing full answers"
- "Amazon codewhisperer outputs blank response"
- "Amazon codewhisperer vs amazon codewhisperer comparison"
- "Are ai apps safe"